Overview
When using STEP Tools® software in MFC applications, you are likely to be writing a GUI, rather than a command-line application, so you may want to capture the warning and error messages from STEP files and display them in a special way. Also, you will need to fix a debugging macro in code generated by Visual Studio wizards.
Conflict with Debug New Macro
If you create an MFC project with Visual Studio wizards, the start of generated .CPP files will have the debugging preprocessor macros shown below. These macros replace every call to new with DEBUG_NEW:
#ifdef _DEBUG #define new DEBUG_NEW <-- This causes problems! #undef THIS_FILE static char THIS_FILE[] = __FILE__; #endif
DEBUG_NEW is itself a macro that calls a special debug version of the new operator with extra filename and line number arguments. This debug version is specific to CObject classes and when called on STEP Tools® C++ classes (which define their own new/pnew operators), the extra arguments cause compiler syntax errors.
To correct this, just comment out the macro that replaces every call to new:
// CORRECTED VERSION #ifdef _DEBUG // define new DEBUG_NEW <-- Commented out #undef THIS_FILE static char THIS_FILE[] = __FILE__; #endif
You can still call DEBUG_NEW explicitly on CObjects if you want to test for memory allocation. In non-debug configurations, the this macro is just defined as the normal new operator. See the Windows AFX.H header file for the exact definitions.
Log Window for Error Reporting
ROSE applications normally print status and debugging messages to stdout, but GUI applications do not have a console, so they will not be seen. You can provide your own message handling as described below, but STEP Tools® software also contains the rose_logwin extension that provides a window to hold a cumulative log of status messages. The messages can be cleared, copied, and saved, and the window can be dismissed when not needed.
Simply include rose_logwin.h and call rose_logwin_init() at the start of your program to register the message window. You must also link against the rose_logwin.lib library.
After this call, all of the ROSE messages are redirected to the window. No other changes are needed. Calling rose_logwin_wait() at the end of your program will block until the user dismisses the message box. This gives the user a chance to view the messages, particularly in short-lived programs.
#include <rose.h> #include <rose_logwin.h> int main (int argc, char ** argv) { rose_logwin_init(); /* your code */ rose_logwin_wait(); }
The rose_logwin extension provides its own Windows message pump in a dedicated thread, and can be used by MFC, raw Windows API, or even console applications. The screendump below shows the message window and some diagnostic messages from a sample application.
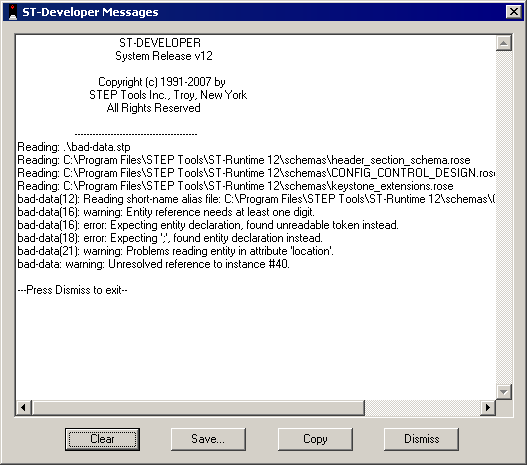
The roselog DLL must be in your path for the log window to appear. It is installed in the STEP Tools® software bin directory, which should be in your path. If the DLL cannot be found, rose_logwin_init() will return zero and the default error handling used.